Chapter 7 Supplementary material
7.1 Exporting SpatRaster to NetCDF Data
When working on a project, you will often need to use the same SpatRaster over and over again. Hence, its convenient to store the model outputs as NetCDFs which can be imported as SpatRaster later for further analysis. Here we will look at the method to export SpatRaster to NetCDF.
Expert Note: a) NetCDF write speeds are significantly slower on cloud platforms like GoogleDrive or OneDrive. Hence, its recommended to export NetCDF files to local disk for faster write speeds. b) Before writing NetCDF file, convert your list of SpatRaster (if any) to one Multi-layer SpatRaster for faster write speed.
library(ncdf4)
rb=rast("./SampleData-master/SMAP_L3_USA.nc")
r=rb[[1]] # raster taken from a first layer of a stack
xy<-xyFromCell(r,1:length(r)) #matrix of logitudes (x) and latitudes(y)
lon=unique(xy[,1])
lat=unique(xy[,2])
# first we write the netcdf file to disk
terra::writeCDF(rb, #SpatRaster
file.path(tempdir(), "test.nc"), #Output filename
overwrite=TRUE,
varname="soil_moisture",
unit="[m3/m3]",
longname="SMAPL3-V7 SM, 2-day interpolated, 36KM",
zname='time')
7.2 Updating R using RStudio
The operations in this tutorial are based on R version 4.1.1- Kick Things
and version 4.0.3 - Bunny-Wunnies Freak Out
. If necessary, update R from Rstudio using the updateR
function from the installr
package.
install.packages("installr")
library(installr)
updateR(keep_install_file=TRUE)
7.3 Changing Temp files for large storage
When processing large rasters/ vectors, you may run out of storage space due to large size of temporary files being generated during processing. Changing temp directory to an external disk with larger storage helps in such case. Follow this discussion for more details: https://stackoverflow.com/questions/17107206/change-temporary-directory.
7.4 Resampling with rgdalwarp
When processing high resolution rasters, you may run out of storage space when using the terra::resample()
. One way to get around it is by using rgdalwarp()
. It is an R wrapper for the ‘gdalwarp’ function that is part of the Geospatial Data Abstraction Library (GDAL), and provides utility for reprojecting rasters into any valid projection. For more details read: https://www.rdocumentation.org/packages/gdalUtils/versions/2.0.3.2/topics/gdalwarp.
The following is an example for resampling MODIS ET (500m) data to 25km resolution.
library(rgdal); library(gdalUtils)
###Resampling with gdalwarp():
#Run the code for the year:
for (year_id in year_first:year_last) {
#Create a date/doy list:
Filenames_tif <- list.files("~/.../MODIS_ET", full.names = F)
Files_subset <- str_subset(Filenames_tif, str_c("_doy",year_id))
#Extract Dates from file names
doy_list <- str_extract(Files_subset, str_sub(Files_subset, 30, 32))
for (doyRun in seq_along(doy_list)) {
#Resample the data
Resampled_Raster<- gdalwarp(
#source file name
srcfile = str_c('~/.../MCD15A2H.061_Lai_500m_doy',year_id,doy_list[doyRun],'_aid0001.tif'),
#destination file name
dstfile = str_c('~/.../MCD15A2H.061_Lai_25km_doy',year_id,doy_list[doyRun],'_aid0001.tif'),
srcnodata = c("249":"255"), #set nodata values
tr = c(0.25, 0.25), #output file resolution: Resampling to 0.25x0.25 degrees (~25km)
te = c(-180,-90,180,90), #georeferenced extents of output file
overwrite=TRUE,
output_Raster = TRUE)
}
}
Follow Part 1: Data Visualization with R for a refresher on ggplot2, wordcloud, OfficeR and text mining.
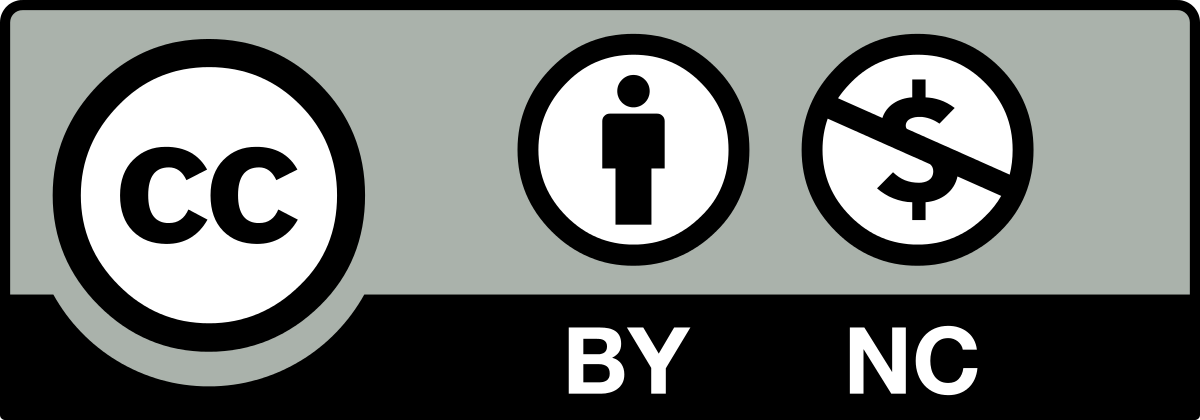